_This is a cross-post of an _article over on the Canadian Developer Connection.
I recently took on the somewhat daunting task of giving a legacy ASP.NET application a major facelift. At first, I thought this would be an easy task. All I need to do is update the CSS, right?
In reality, this was an application with 8 years of history and at least 10 different CSS files that had all evolved separately.
It was at this point that I decided some CSS refactoring was required in order to successfully pull off this facelift.
Enter LESS
I remembered attending a conference session about LESS and I thought it might help to simplify my CSS.
LESS is an extension to CSS that adds some features that can help make your CSS files a little easier to manage. Typically, LESS is compiled to CSS and the browser doesn’t even know that LESS was used in the background. No browser extensions or client side libraries are needed to use LESS.
One of the most basic LESS features is variables, which allow you to control commonly used values in a single location. For me, this was a great starting point. Since I knew I would be spending some time tweaking the color scheme for the application, it would be great if I could define all my colors in one place and reference those colors in the various CSS files in my application.
Getting started with LESS in Visual Studio
If you have the Web Essentials extension installed (and you should), getting started with LESS is extremely easy. First, rename your .css files to .less. Next, open each .less file and click Save. Web Essentials will create a .css and a .min.css file nested below the new .less file. Any time you make a change to your LESS file, Web Essentials will regenerate the .css and .min.css files.
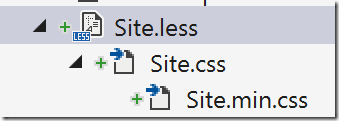
You will also notice that when you open the .less file the source is split vertically.
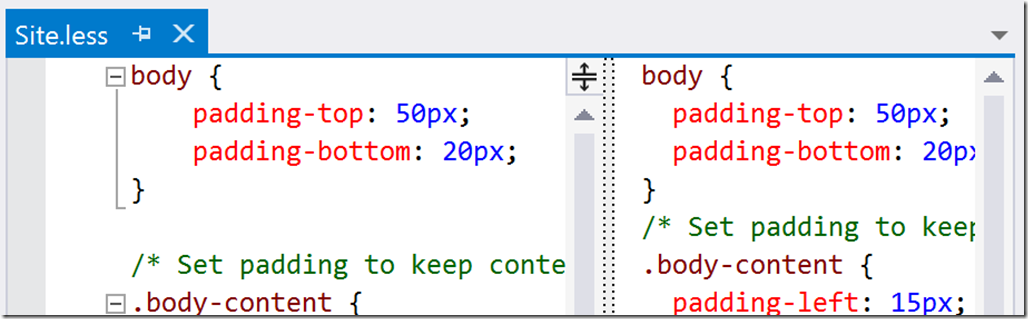
The code on the left is your LESS code. The code on the right is a preview of the resulting CSS code that Web Essential will generate for you. Since we have not made use of any of the LESS features, the code on the left should be the same as the code on the right.
Defining Color Variables
You can define variables using the syntax @variable-name: variableValue;
Start by adding a variable @primary-color: at the top of your main LESS file. If you start to type a hex color be adding #, a Web Essentials popup will appear listing all the colors found in this file.
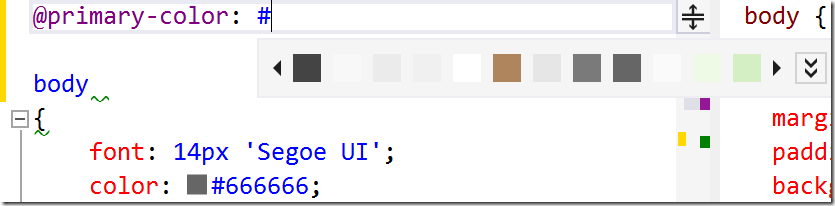
Select the color that represents your primary color. Now replace all other occurrences of that color with @primary-color.
When Web Essentials compiles the .less file to .css, @primary-color will be replaced with the value assigned to that variable in the .less file.
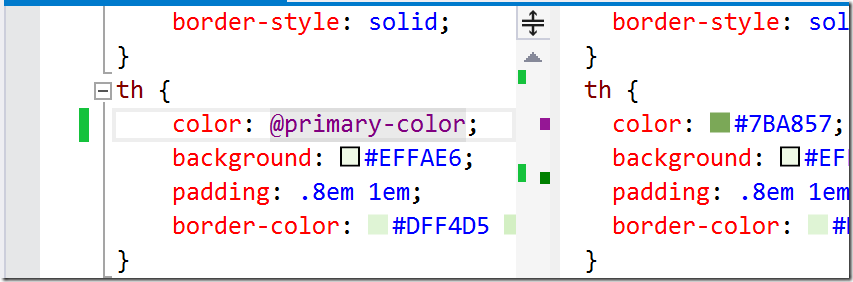
Now repeat this process with all the colors in your less file. Eventually, you should end up with a concise theme defined at the top of the file.
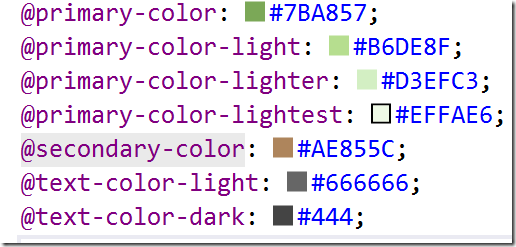
If you want to make changes to your color theme, simply change these color variables and the changes will auto-magically appear throughout the resulting CSS file.
Adding Functions
We can take this a step further and make use of LESS functions. Instead of defining 4 different shades of green for our various shades of primary colors, we can use a function to programmatically lighten the primary color as follows:
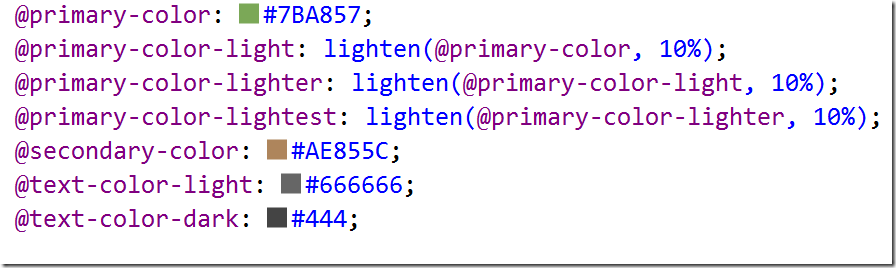
Now this LESS

compiles to this CSS:
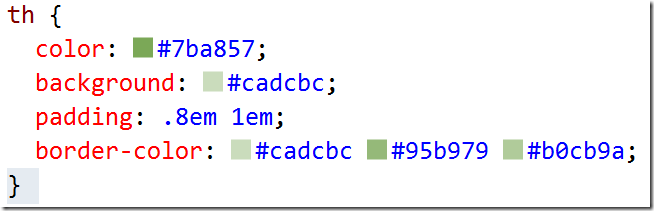
Now, If you want to change your primary color from green to blue, you can change the single @primary-color variable and all the various shades of green will change to shades of blue.
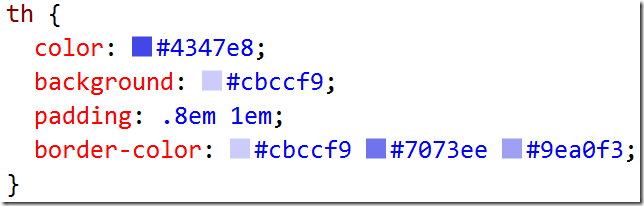
If like me, your CSS was split across numerous files, you can also extract your color variables into a separate LESS file and reference those colors from other files.
To extract the colors to a separate file, select the lines containing all the color variables, right click and select Web Essentials –> Extract to file. Name the file colors.less.
In the original less file, add _import “colors.less”; _to the top of the file.

Next, import the colors.less file into all your other LESS files and you will be able to reference the color variables from all your LESS files.
Now, you have a single place to change the colors everywhere in your application.
Web Essentials Settings
Web Essentials has some settings for how it supports LESS. You can access Web Essentials settings by selecting Tools –> Options from the Visual Studio menu, then selecting Web Essentials –> LESS.
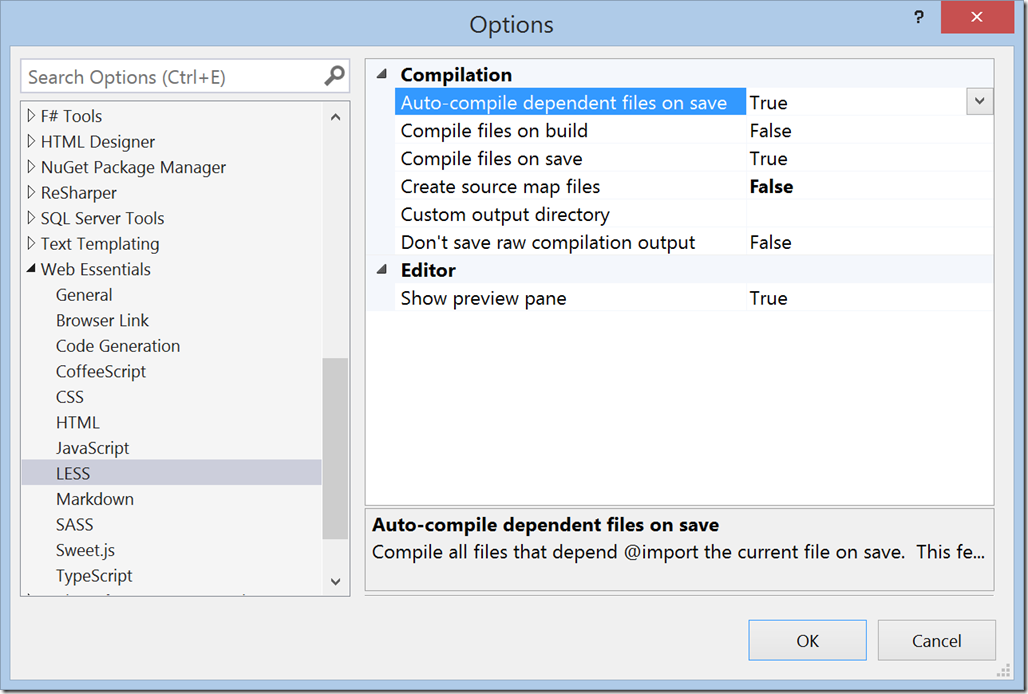
You can choose to compile to CSS on build instead of on save. You can also choose to hide the preview pane.
Summary
We have really only scratched the surface of what LESS can do for you. For me, it was a huge help when trying to apply some more modern styling to a somewhat dated web application.
Visit the LESS website to learn more about other features that can help you. I found Variables, Mixins, various built in Functions to be very useful.